반응형
도트매트릭스는 Matrix(행렬)형태로 도트(Dot)모양의 LED를 연결한 제품입니다. 각 도트의 LED를 제어하여 어떤 모양이나 글자를 나타낼 수 있습니다.
여기서 우리가 사용할 LED는 "8x8 도트 매트릭스 LED"로 가로 8개, 세로 8개의 행렬로 이루어져 있으며, 총 64개의 LED(8x8)의 격자형으로 구성해 놓은 MAX8219 LED 장치입니다.
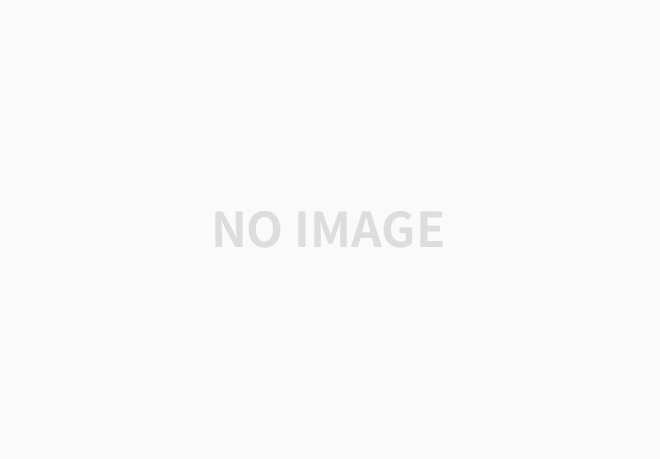
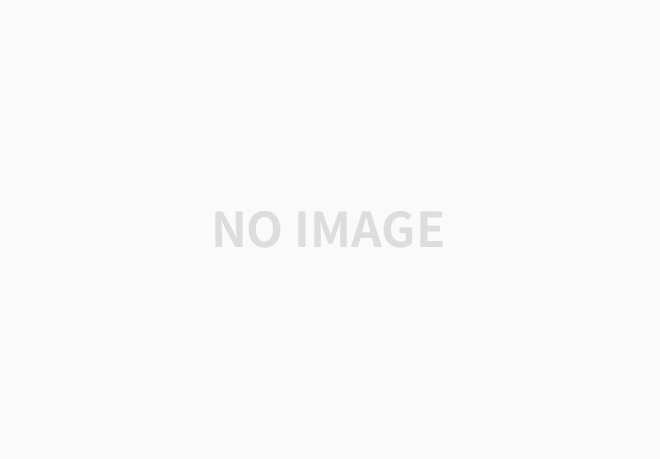
1) LED 하나씩 켜기
- 회로도
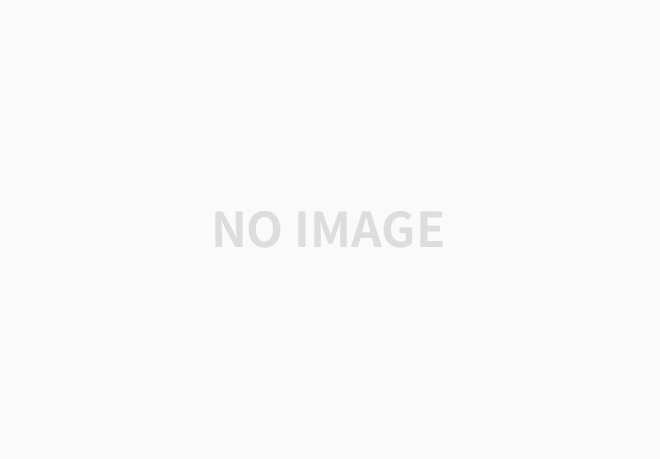
- 라이브러리 설치하기
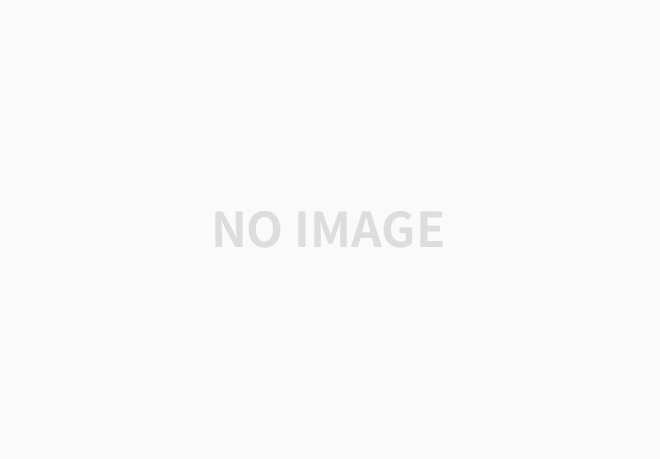
- 소스코드
/*
8x8 도트매트릭스 연결한 후 각 LED를 제어해봅시다.
*/
#include "LedControl.h"
// 아두이노보드의 12,11,10핀을 사용하고, 1개의 8x8 도트매트릭스를 제어하는 도트매트릭스 객체를 생성합니다.
LedControl lc = LedControl(12,10,11,1);
void setup(){
// 도트매트릭스의 절전모드를 꺼줍니다.
lc.shutdown(0,false);
// 도트매트릭스의 LED 밝기를 8로 지정해 줍니다.(0~15)
lc.setIntensity(0,8);
// 도트매트릭스의 LED를 초기화 해줍니다.
lc.clearDisplay(0);
}
void loop(){
// 도트매트릭스의 LED를 첫번째 부터 1개씩 차례대로 켜줍니다.
for (int row=0; row<8; row++) {
for (int col=0; col<8; col++) {
lc.setLed(0,col,row,true);
delay(25);
}
}
// 도트매트릭스의 LED를 첫번째 부터 1개씩 차례대로 꺼줍니다.
for (int row=0; row<8; row++) {
for (int col=0; col<8; col++) {
lc.setLed(0,col,row,false);
delay(25);
}
}
}
- 결과
2) 하트 그리기-1
- 소스코드
unsigned char i;
unsigned char j;
int Max7219_pinCLK = 10;
int Max7219_pinCS = 11;
int Max7219_pinDIN = 12;
unsigned char disp1[19][8]={
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // Heart Pattern
0x00, 0x00, 0x00, 0x00, 0x40, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x40, 0x40, 0x00, 0x00, 0x00,
0x00, 0x00, 0x80, 0x40, 0x40, 0x00, 0x00, 0x00,
0x00, 0x80, 0x80, 0x40, 0x40, 0x00, 0x00, 0x00,
0x40, 0x80, 0x80, 0x40, 0x40, 0x00, 0x00, 0x00,
0x60, 0x80, 0x80, 0x40, 0x40, 0x00, 0x00, 0x00,
0x60, 0x90, 0x80, 0x40, 0x40, 0x00, 0x00, 0x00,
0x60, 0x90, 0x88, 0x40, 0x40, 0x00, 0x00, 0x00,
0x60, 0x90, 0x88, 0x44, 0x40, 0x00, 0x00, 0x00,
0x60, 0x90, 0x88, 0x44, 0x44, 0x00, 0x00, 0x00,
0x60, 0x90, 0x88, 0x44, 0x44, 0x08, 0x00, 0x00,
0x60, 0x90, 0x88, 0x44, 0x44, 0x08, 0x10, 0x00,
0x60, 0x90, 0x88, 0x44, 0x44, 0x08, 0x10, 0x20,
0x60, 0x90, 0x88, 0x44, 0x44, 0x08, 0x10, 0x60,
0x60, 0x90, 0x88, 0x44, 0x44, 0x08, 0x90, 0x60,
0x60, 0x90, 0x88, 0x44, 0x44, 0x88, 0x90, 0x60, // Heart Pattern
};
void Write_Max7219_byte(unsigned char DATA) {
unsigned char i;
digitalWrite(Max7219_pinCS,LOW);
for(i=8;i>=1;i--) {
digitalWrite(Max7219_pinCLK,LOW);
digitalWrite(Max7219_pinDIN,DATA&0x80);
DATA = DATA<<1;
digitalWrite(Max7219_pinCLK,HIGH);
}
}
void Write_Max7219(unsigned char address,unsigned char dat){
digitalWrite(Max7219_pinCS,LOW);
Write_Max7219_byte(address);
Write_Max7219_byte(dat);
digitalWrite(Max7219_pinCS,HIGH);
}
void Init_MAX7219(void){
Write_Max7219(0x09, 0x00);
Write_Max7219(0x0a, 0x03);
Write_Max7219(0x0b, 0x07);
Write_Max7219(0x0c, 0x01);
Write_Max7219(0x0f, 0x00);
}
void setup(){
pinMode(Max7219_pinCLK,OUTPUT);
pinMode(Max7219_pinCS,OUTPUT);
pinMode(Max7219_pinDIN,OUTPUT);
delay(50);
Init_MAX7219();
}
void loop(){
for(j=0;j<19;j++) {
for(i=1;i<9;i++)
Write_Max7219(i,disp1[j][i-1]);
delay(500);
}
}
- 결과
3) 하트 그리기-2
#include "LedControl.h" // 도트매트릭스 라이브러리
// 도트매트릭스 연결핀
LedControl lc = LedControl(12, 10, 11, 1); // (DIN,CLK,CS,number)
// 하트 모양의 배열 데이터
byte heart[] ={
B01100110,
B11111111,
B11111111,
B11111111,
B01111110,
B00111100,
B00011000,
B00000000
};
void setup() {
lc.shutdown(0, false);
lc.setIntensity(0, 5); // LED 도트매트릭스 밝기
lc.clearDisplay(0); // LED 도트매트릭스 모두 지우기
}
void loop() {
showLED(heart, 1); // 하트모양 보임
delay(1000); // 1초 기다리기
showLED(heart, 0); // 모두 지움
delay(1000); // 1초 기다리기
}
// 도트매트릭스 제어용 함수
void showLED(byte arr[], int a)
{
if (a == 1) {
for (int i = 0; i < 8; i++)
{
lc.setRow(0, i, arr[i]);
}
} else {
for (int i = 0; i < 8; i++)
{
lc.setRow(0, i, B00000000);
}
}
}
-결과
[모양만들기]
LED Matrix(8x8) Generator : https://xantorohara.github.io/led-matrix-editor/
LED Matrix Editor
Overview LED Matrix Editor - is online tool for editing and creating animations for 8x8 LED matrices. It is free and easy to use. Usage Click on LED to toggle single item Click on row or column numbers to toggle whole row or column Click on icon to clear/f
xantorohara.github.io
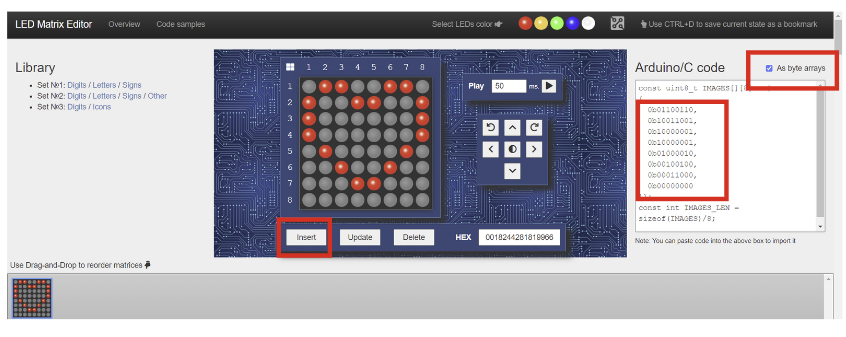
- 소스코드
#include "LedControl.h" // 도트매트릭스 라이브러리
// 도트매트릭스 연결핀
LedControl lc = LedControl(12, 10, 11, 1); // (DIN,CLK,CS,number)
// 하트 모양의 배열 데이터
byte heart[] ={
B01100110,
B11111111,
B11111111,
B11111111,
B01111110,
B00111100,
B00011000,
B00000000
};
byte heart_1[] ={ //새로 만든 하트 모양 데이터 값
0b01100110,
0b10011001,
0b10000001,
0b10000001,
0b01000010,
0b00100100,
0b00011000,
0b00000000
};
void setup() {
lc.shutdown(0, false);
lc.setIntensity(0, 5); // LED 도트매트릭스 밝기
lc.clearDisplay(0); // LED 도트매트릭스 모두 지우기
}
void loop() {
showLED(heart, 1); // 하트모양 보임
delay(500);
showLED(heart, 0); // 모두 지움
delay(500);
showLED(heart_1, 1); // 하트모양_1 보임
delay(500);
showLED(heart_1, 0); // 모두 지움
delay(500);
}
// 도트매트릭스 제어용 함수
void showLED(byte arr[], int a)
{
if (a == 1) {
for (int i = 0; i < 8; i++)
{
lc.setRow(0, i, arr[i]);
}
} else {
for (int i = 0; i < 8; i++)
{
lc.setRow(0, i, B00000000);
}
}
}
-결과
4) 글자 나타내기
- 라이브러리 설치하기
첨부파일을 다운로드하여 라이브러리를 설치합니다.
LED_matrix_master.zip
0.01MB
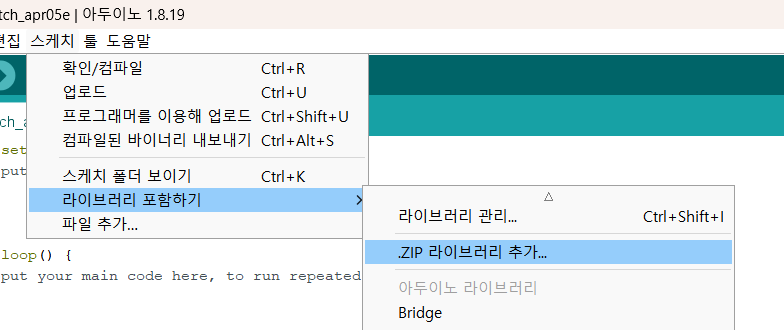
- 소스코드
#include "LedControlMS.h"
//pin 12 is connected to the DataIn
//pin 11 is connected to LOAD
// pin 10 is connected to the CLK
#define NBR_MTX 1 //number of matrices attached is one
LedControl lc=LedControl(12,10, 11, NBR_MTX);//
void setup(){
for (int i=0; i< NBR_MTX; i++) {
lc.shutdown(i,false);
/* Set the brightness to a medium values */
lc.setIntensity(i,8);
/* and clear the display */
lc.clearDisplay(i);
delay(500);
}
}
void loop() {
lc.writeString(0,"IAMHAPPY");//sending characters to display
lc.clearAll();//clearing the display
delay(1000);
}
- 결과
4) 도트 매트릭스 여러개 사용하기
- 회로도
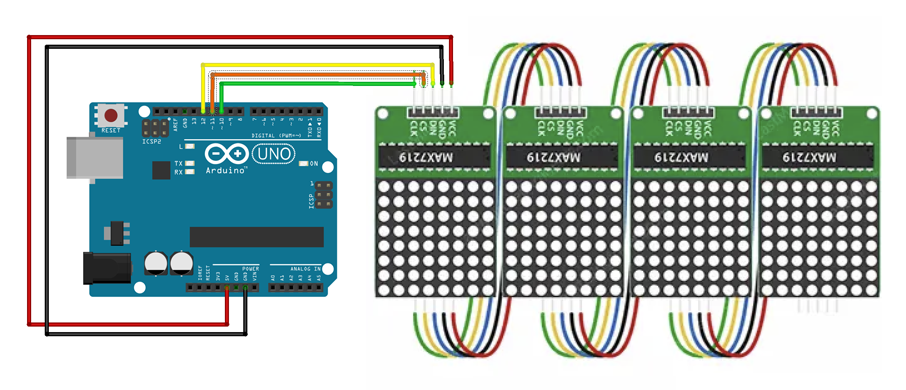
= 소스코드
//We always have to include the library
#include "LedControl.h"
LedControl lc = LedControl(12, 10, 11, 5);
/* we always wait a bit between updates of the display */
unsigned long delaytime = 50;
void setup() {
lc.shutdown(0, false);
lc.setIntensity(0, 8);
lc.clearDisplay(0);
lc.shutdown(1, false);
lc.setIntensity(1, 8);
lc.clearDisplay(1);
lc.shutdown(2, false);
lc.setIntensity(2, 8);
lc.clearDisplay(2);
lc.shutdown(3, false);
lc.setIntensity(3, 8);
lc.clearDisplay(3);
lc.shutdown(4, false);
lc.setIntensity(4, 8);
lc.clearDisplay(4);
}
void loop() {
// for (int row = 0; row < 40; row++) {
// for (int col = 0; col < 8; col++) {
// lc.setLed(row / 8, row % 8, col, true) ;
// delay(delaytime) ;
// }
// }
//
// lc.clearDisplay(0) ;
// lc.clearDisplay(1) ;
// lc.clearDisplay(2) ;
// lc.clearDisplay(3) ;
// lc.clearDisplay(4) ;
for (int col = 0; col < 8; col++) {
for (int row = 0; row < 40; row++) {
lc.setLed(row / 8, row % 8, col, true) ;
delay(delaytime) ;
}
}
lc.clearDisplay(0) ;
lc.clearDisplay(1) ;
lc.clearDisplay(2) ;
lc.clearDisplay(3) ;
lc.clearDisplay(4) ;
}
5) 문장 슬라이딩 표현하기
- 아래 첨부파일을 다운로드하여 라이브러리 추가
MaxMatrix.zip
0.00MB
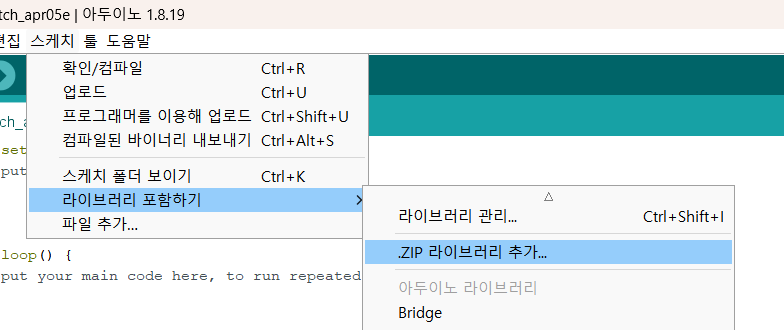
#include <MaxMatrix.h>
#include <avr/pgmspace.h>
PROGMEM const unsigned char CH[] = {
3, 8, B00000000, B00000000, B00000000, B00000000, B00000000, // space
1, 8, B01011111, B00000000, B00000000, B00000000, B00000000, // !
3, 8, B00000011, B00000000, B00000011, B00000000, B00000000, // "
5, 8, B00010100, B00111110, B00010100, B00111110, B00010100, // #
4, 8, B00100100, B01101010, B00101011, B00010010, B00000000, // $
5, 8, B01100011, B00010011, B00001000, B01100100, B01100011, // %
5, 8, B00110110, B01001001, B01010110, B00100000, B01010000, // &
1, 8, B00000011, B00000000, B00000000, B00000000, B00000000, // '
3, 8, B00011100, B00100010, B01000001, B00000000, B00000000, // (
3, 8, B01000001, B00100010, B00011100, B00000000, B00000000, // )
5, 8, B00101000, B00011000, B00001110, B00011000, B00101000, // *
5, 8, B00001000, B00001000, B00111110, B00001000, B00001000, // +
2, 8, B10110000, B01110000, B00000000, B00000000, B00000000, // ,
4, 8, B00001000, B00001000, B00001000, B00001000, B00000000, // -
2, 8, B01100000, B01100000, B00000000, B00000000, B00000000, // .
4, 8, B01100000, B00011000, B00000110, B00000001, B00000000, // /
4, 8, B00111110, B01000001, B01000001, B00111110, B00000000, // 0
3, 8, B01000010, B01111111, B01000000, B00000000, B00000000, // 1
4, 8, B01100010, B01010001, B01001001, B01000110, B00000000, // 2
4, 8, B00100010, B01000001, B01001001, B00110110, B00000000, // 3
4, 8, B00011000, B00010100, B00010010, B01111111, B00000000, // 4
4, 8, B00100111, B01000101, B01000101, B00111001, B00000000, // 5
4, 8, B00111110, B01001001, B01001001, B00110000, B00000000, // 6
4, 8, B01100001, B00010001, B00001001, B00000111, B00000000, // 7
4, 8, B00110110, B01001001, B01001001, B00110110, B00000000, // 8
4, 8, B00000110, B01001001, B01001001, B00111110, B00000000, // 9
2, 8, B01010000, B00000000, B00000000, B00000000, B00000000, // :
2, 8, B10000000, B01010000, B00000000, B00000000, B00000000, // ;
3, 8, B00010000, B00101000, B01000100, B00000000, B00000000, // <
3, 8, B00010100, B00010100, B00010100, B00000000, B00000000, // =
3, 8, B01000100, B00101000, B00010000, B00000000, B00000000, // >
4, 8, B00000010, B01011001, B00001001, B00000110, B00000000, // ?
5, 8, B00111110, B01001001, B01010101, B01011101, B00001110, // @
4, 8, B01111110, B00010001, B00010001, B01111110, B00000000, // A
4, 8, B01111111, B01001001, B01001001, B00110110, B00000000, // B
4, 8, B00111110, B01000001, B01000001, B00100010, B00000000, // C
4, 8, B01111111, B01000001, B01000001, B00111110, B00000000, // D
4, 8, B01111111, B01001001, B01001001, B01000001, B00000000, // E
4, 8, B01111111, B00001001, B00001001, B00000001, B00000000, // F
4, 8, B00111110, B01000001, B01001001, B01111010, B00000000, // G
4, 8, B01111111, B00001000, B00001000, B01111111, B00000000, // H
3, 8, B01000001, B01111111, B01000001, B00000000, B00000000, // I
4, 8, B00110000, B01000000, B01000001, B00111111, B00000000, // J
4, 8, B01111111, B00001000, B00010100, B01100011, B00000000, // K
4, 8, B01111111, B01000000, B01000000, B01000000, B00000000, // L
5, 8, B01111111, B00000010, B00001100, B00000010, B01111111, // M
5, 8, B01111111, B00000100, B00001000, B00010000, B01111111, // N
4, 8, B00111110, B01000001, B01000001, B00111110, B00000000, // O
4, 8, B01111111, B00001001, B00001001, B00000110, B00000000, // P
4, 8, B00111110, B01000001, B01000001, B10111110, B00000000, // Q
4, 8, B01111111, B00001001, B00001001, B01110110, B00000000, // R
4, 8, B01000110, B01001001, B01001001, B00110010, B00000000, // S
5, 8, B00000001, B00000001, B01111111, B00000001, B00000001, // T
4, 8, B00111111, B01000000, B01000000, B00111111, B00000000, // U
5, 8, B00001111, B00110000, B01000000, B00110000, B00001111, // V
5, 8, B00111111, B01000000, B00111000, B01000000, B00111111, // W
5, 8, B01100011, B00010100, B00001000, B00010100, B01100011, // X
5, 8, B00000111, B00001000, B01110000, B00001000, B00000111, // Y
4, 8, B01100001, B01010001, B01001001, B01000111, B00000000, // Z
2, 8, B01111111, B01000001, B00000000, B00000000, B00000000, // [
4, 8, B00000001, B00000110, B00011000, B01100000, B00000000, // \ backslash
2, 8, B01000001, B01111111, B00000000, B00000000, B00000000, // ]
3, 8, B00000010, B00000001, B00000010, B00000000, B00000000, // hat
4, 8, B01000000, B01000000, B01000000, B01000000, B00000000, // _
2, 8, B00000001, B00000010, B00000000, B00000000, B00000000, // `
4, 8, B00100000, B01010100, B01010100, B01111000, B00000000, // a
4, 8, B01111111, B01000100, B01000100, B00111000, B00000000, // b
4, 8, B00111000, B01000100, B01000100, B00101000, B00000000, // c
4, 8, B00111000, B01000100, B01000100, B01111111, B00000000, // d
4, 8, B00111000, B01010100, B01010100, B00011000, B00000000, // e
3, 8, B00000100, B01111110, B00000101, B00000000, B00000000, // f
4, 8, B10011000, B10100100, B10100100, B01111000, B00000000, // g
4, 8, B01111111, B00000100, B00000100, B01111000, B00000000, // h
3, 8, B01000100, B01111101, B01000000, B00000000, B00000000, // i
4, 8, B01000000, B10000000, B10000100, B01111101, B00000000, // j
4, 8, B01111111, B00010000, B00101000, B01000100, B00000000, // k
3, 8, B01000001, B01111111, B01000000, B00000000, B00000000, // l
5, 8, B01111100, B00000100, B01111100, B00000100, B01111000, // m
4, 8, B01111100, B00000100, B00000100, B01111000, B00000000, // n
4, 8, B00111000, B01000100, B01000100, B00111000, B00000000, // o
4, 8, B11111100, B00100100, B00100100, B00011000, B00000000, // p
4, 8, B00011000, B00100100, B00100100, B11111100, B00000000, // q
4, 8, B01111100, B00001000, B00000100, B00000100, B00000000, // r
4, 8, B01001000, B01010100, B01010100, B00100100, B00000000, // s
3, 8, B00000100, B00111111, B01000100, B00000000, B00000000, // t
4, 8, B00111100, B01000000, B01000000, B01111100, B00000000, // u
5, 8, B00011100, B00100000, B01000000, B00100000, B00011100, // v
5, 8, B00111100, B01000000, B00111100, B01000000, B00111100, // w
5, 8, B01000100, B00101000, B00010000, B00101000, B01000100, // x
4, 8, B10011100, B10100000, B10100000, B01111100, B00000000, // y
3, 8, B01100100, B01010100, B01001100, B00000000, B00000000, // z
3, 8, B00001000, B00110110, B01000001, B00000000, B00000000, // {
1, 8, B01111111, B00000000, B00000000, B00000000, B00000000, // |
3, 8, B01000001, B00110110, B00001000, B00000000, B00000000, // }
4, 8, B00001000, B00000100, B00001000, B00000100, B00000000, // ~
};
int data = 12; // DIN pin of MAX7219 displays
int load = 11 ; // CS pin of MAX7219 displays
int clock = 10; // CLK pin of MAX7219 displays
int scrollSpeed = 150; // speed of scrolling text, lower is faster
int maxInUse = 5; //Number of MAX7219 displays
MaxMatrix m(data, load, clock, maxInUse); // define Library
byte buffer[10];
char inData[64] = "Believe in yourself!"; // LED에 나올 문장 (max 64 bytes)
char inChar; // Where to store the character read
byte index = 0; // Index into array; where to store the character
void setup(){
m.init(); // init display
m.setIntensity(7); // LED light intensity from 0 (dimmest) to 15
Serial.begin(9600); // init serial connection
}
void loop(){
// Get input from serial connection
index=0;
while(Serial.available() > 0) { // Don't read unless there is data
if(index < 63) { // One less than the size of the array
inChar = Serial.read(); // Read a character
Serial.write(inChar);
inData[index] = inChar; // Store it
index++; // Increment where to write next
inData[index] = '\0'; // Null terminate the string
}
}
byte c;
delay(100);
m.shiftLeft(false, true);
printStringWithShift(inData, scrollSpeed); // Send scrolling Text
clearLCD(maxInUse, scrollSpeed);
}
// Put extracted character on Display
void printCharWithShift(char c, int shift_speed){
if (c < 32) return;
c -= 32;
memcpy_P(buffer, CH + 7*c, 7);
m.writeSprite(maxInUse*8, 0, buffer);
m.setColumn(maxInUse*8 + buffer[0], 0);
for (int i=0; i<buffer[0]+1; i++) {
delay(shift_speed);
m.shiftLeft(false, false);
}
}
// Extract characters from Scrolling text
void printStringWithShift(char* s, int shift_speed){
while (*s != 0){
printCharWithShift(*s, shift_speed);
s++;
}
}
// Add trailing space to clear the display
void clearLCD(int maxInUse, int shift_speed){
for (int i=0; i<maxInUse*8; i++)
{
m.setColumn((maxInUse*8), 0);
delay(shift_speed);
m.shiftLeft(false, false);
}
}
- 결과
- 세로로 문장 슬라이딩 하기
//We always have to include the library
#include "LedControl.h"
LedControl lc = LedControl(12, 10, 11, 5);
byte mat48[5][8] ={
{ 0x00, 0x22, 0x2a, 0x6a, 0x26, 0x22, 0x22, 0x00},//사
{0x70, 0x48, 0x36, 0x22, 0x6e, 0x28, 0x2e, 0x00},//랑
{0x00, 0xa4, 0xaa, 0xee, 0xa0, 0xae, 0xa4, 0x00},//해
{0x00, 0x7c, 0x28, 0x38, 0x44, 0x44, 0x38, 0x00}, //요
{0x00, 0x00, 0x10, 0x38, 0x7c, 0x7c, 0x28, 0x00} //하트
};
/* we always wait a bit between updates of the display */
unsigned long delayTime = 50;
void setup() {
lc.shutdown(0, false);
lc.shutdown(1, false);
lc.shutdown(2, false);
lc.shutdown(3, false);
lc.shutdown(4, false);
lc.setIntensity(0, 5);
lc.setIntensity(1, 5);
lc.setIntensity(2, 5);
lc.setIntensity(3, 5);
lc.setIntensity(4, 5);
lc.clearDisplay(0);
lc.clearDisplay(1);
lc.clearDisplay(2);
lc.clearDisplay(3);
lc.clearDisplay(4);
}
void loop() {
for(int i=0;i<5;i++){
display_Text(mat48[i],4-i);
}
delay(delayTime);
shift_Left_5MAT(&mat48[0][0]);
}
void display_Text(byte *bt, int num){
for(int i=0;i<8;i++){
lc.setRow(num, i, *(bt+i));
}
}
void shift_Left_5MAT(byte *bt){
byte b01=0x01;
byte b80=0x80;
int flag[5][8]={{0,0,0,0,0,0,0,0},{0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0}, {0,0,0,0,0,0,0,0}, {0,0,0,0,0,0,0,0}};
for (int m=0;m<5;m++){
for (int i=0;i<8;i++){
if (*(bt+m*8+i)&b80) flag[m][i]=1;
*(bt+m*8+i)<<=1;
}
}
for (int m=0;m<5;m++){
for (int i=0;i<8;i++){
if (flag[m+1][i]==1) *(bt+m*8+i)|=b01;
}
}
for (int i=0;i<8;i++){
if (flag[0][i]==1) *(bt+4*8+i)|=b01;
}
for (int m=0;m<5;m++){
for (int i=0;i<8;i++) flag[m][i]=0;
}
}
- 결과
반응형
'피지컬컴퓨팅 > 아두이노' 카테고리의 다른 글
#인공지능 AI 활용 - openCV를 활용하여 아두이노 제어(얼굴인식 도어락) (0) | 2024.03.29 |
---|---|
RFID 모듈 활용하기(도어락 만들기) (0) | 2024.03.26 |
FND 4 digit 7 segment 활용하기 (0) | 2024.03.26 |
센서 활용하기-조도센서, 물높이 센서, 기울기 센서 등 (0) | 2024.03.25 |
I2C LCD 활용하기(센서 측정 값 나타내기-초음파센서, 온습도 센서) (0) | 2024.03.21 |