반응형
DS1302는 댈러스가 출시한 슬라이딩 충전시계 칩으로, 매우 낮은 전력으로 작동하도록 설계되어 1µW 미만의 데이터와 클럭 정보를 유지할 수 있습니다. DS1302는 RTC(Real Time Clock) 시간을 저장하고 출력하는 장치로, 일반적인 디지털 시계 등에 많이 사용됩니다. DS1302는 CR2032 건전지를 사용해 전력을 공급받습니다. 배터리의 수명이 끝났거나 배터리가 모듈에서 분리되는 경우 DS1302 칩에 기록된 시간은 초기화(리셋)됩니다.
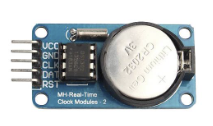
DS1302를 사용하려면 라이브러리를 설치 및 추가해야 합니다.
1) 현재 시간 설정하여 출력하기
- RTC에 현재 시간을 설정하여 시리얼 모니터에 출력해 보겠습니다.
회로도
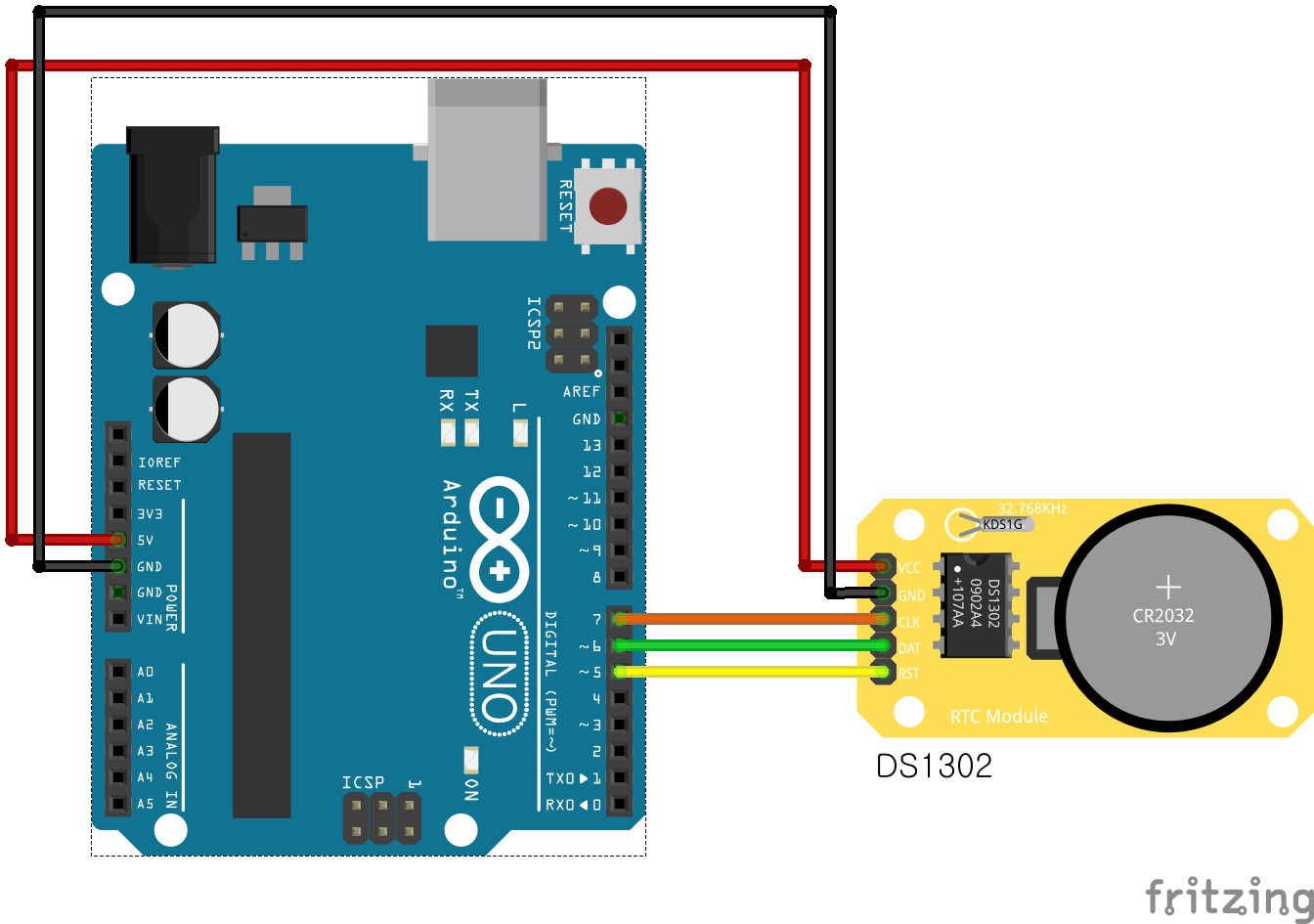
- 라이브러리 설치하기 : 첨부파일을 다운로드한 후 설치합니다.
- 스케치->라이브러리 포함하기->라이브러리 관리
다운로드한 zip파일을 선택하여 라이브러리를 추가합니다.
소스코드
// Example sketch for interfacing with the DS1302 timekeeping chip.
//
// Copyright (c) 2009, Matt Sparks
// All rights reserved.
//
// http://quadpoint.org/projects/arduino-ds1302
#include <stdio.h>
#include <DS1302.h>
namespace {
// Set the appropriate digital I/O pin connections. These are the pin
// assignments for the Arduino as well for as the DS1302 chip. See the DS1302
// datasheet:
//
// http://datasheets.maximintegrated.com/en/ds/DS1302.pdf
const int kCePin = 5; // Chip Enable
const int kIoPin = 6; // Input/Output
const int kSclkPin = 7; // Serial Clock
// Create a DS1302 object.
DS1302 rtc(kCePin, kIoPin, kSclkPin);
String dayAsString(const Time::Day day) {
switch (day) {
case Time::kSunday: return "Sunday";
case Time::kMonday: return "Monday";
case Time::kTuesday: return "Tuesday";
case Time::kWednesday: return "Wednesday";
case Time::kThursday: return "Thursday";
case Time::kFriday: return "Friday";
case Time::kSaturday: return "Saturday";
}
return "(unknown day)";
}
void printTime() {
// Get the current time and date from the chip.
Time t = rtc.time();
// Name the day of the week.
const String day = dayAsString(t.day);
// Format the time and date and insert into the temporary buffer.
char buf[50];
snprintf(buf, sizeof(buf), "%s %04d-%02d-%02d %02d:%02d:%02d",
day.c_str(),
t.yr, t.mon, t.date,
t.hr, t.min, t.sec);
// Print the formatted string to serial so we can see the time.
Serial.println(buf);
}
} // namespace
void setup() {
Serial.begin(9600);
// Initialize a new chip by turning off write protection and clearing the
// clock halt flag. These methods needn't always be called. See the DS1302
// datasheet for details.
rtc.writeProtect(false);
rtc.halt(false);
// Make a new time object to set the date and time.
// Sunday, September 22, 2013 at 01:38:50.
Time t(2024, 4, 5, 8, 38, 50, Time::kFriday);
// Set the time and date on the chip.
rtc.time(t);
}
// Loop and print the time every second.
void loop() {
printTime();
delay(1000);
}
결과
2) LCD 에 날짜시간 나타내기
- RTC에 설정된 현재 시간과 날짜, 요일을 LCD에 표시합니다.
회로도
소스코드
#include <stdio.h>
#include <DS1302.h>
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x27, 16, 2);
namespace {
// DS1302와 연결되는 아두이노 핀 번호
const int kCePin = 5; // Chip Enable
const int kIoPin = 6; // Input/Output
const int kSclkPin = 7; // Serial Clock
// DS1302 오브젝트 생성
DS1302 rtc(kCePin, kIoPin, kSclkPin);
// 요일 코드에 알맞는 문자열 출력
String dayAsString(const Time::Day day) {
switch (day) {
case Time::kSunday: return "SUN";
case Time::kMonday: return "MON";
case Time::kTuesday: return "TUE";
case Time::kWednesday: return "WED";
case Time::kThursday: return "THU";
case Time::kFriday: return "FRI";
case Time::kSaturday: return "SAT";
}
return "(unknown day)";
}
// 날짜, 시간 출력 함수
//void printTime() {
// // 칩에 저장된 시간을 읽어옵니다.
// Time t = rtc.time();
//
// // 요일 코드에 알맞는 문자열 가져오기
// const String day = dayAsString(t.day);
//
// // 날짜와 시간 정보를 임시 버퍼에 형식에 맞추어 담아둡니다.
// char buf[50];
// snprintf(buf, sizeof(buf), "%s %04d-%02d-%02d %02d:%02d:%02d",
// day.c_str(),t.yr, t.mon, t.date,t.hr, t.min, t.sec);
//
// // 임시 버퍼의 내용을 출력합니다.
// Serial.println(buf);
//}
} // namespace
void setup() {
Serial.begin(9600);
lcd.init();
lcd.backlight();
// 새로운 날짜와 시간 정보를 칩에 저장합니다.
// 이 작업은 시간을 맞출때만 사용하며, 평상시에는 주석(//) 처리되어 있어야 합니다.
rtc.writeProtect(false);
rtc.halt(false);
Time t(2024, 4, 5, 14, 49, 00, Time::kTuesday); //현재 날짜와 시간을 설정
rtc.time(t);
}
void loop() {
// printTime();
Time t = rtc.time();
// 요일 코드에 알맞는 문자열 가져오기
const String day = dayAsString(t.day);
// 날짜와 시간 정보를 임시 버퍼에 형식에 맞추어 담아둡니다.
char buf[50];
snprintf(buf, sizeof(buf), "%s %04d-%02d-%02d %02d:%02d:%02d",
day.c_str(),t.yr, t.mon, t.date,t.hr, t.min, t.sec);
// 임시 버퍼의 내용을 출력합니다.
Serial.println(buf);
lcd.setCursor(2,0);
lcd.print(t.yr); lcd.print("/");
lcd.print( t.mon); lcd.print("/");
lcd.print(t.date); lcd.print("/");
lcd.print(day.c_str());
lcd.setCursor(5,1);
lcd.print(t.hr);
lcd.print(":");
lcd.print(t.min);
lcd.print(":");
lcd.print(t.sec);
delay(1000);
lcd.clear();
}
결과
반응형
'메이킹 > 아두이노' 카테고리의 다른 글
Mediapipe로 HandTracking 하여 LED 제어하기 (0) | 2024.04.17 |
---|---|
4x4 키패드 활용하기 (0) | 2024.04.11 |
조이스틱 활용하기(LED 제어하기) (0) | 2024.03.29 |
openCV를 활용하여 아두이노 제어하기(얼굴인식 도어락) (0) | 2024.03.29 |
아두이노와 파이썬 연동하기 (2) | 2024.03.28 |